My lack of maths skill will be my undoing…
I have some normal maps, you know the kinda thing…
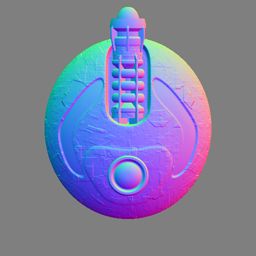
And I have all the code (dot product stuff) that plugs in the float3 that is the light direction, and then gives me a tint I can apply to the final pixel to get a nice pseudo 3D bump map effect. Thats all lovely and works, and is cool. The problem is, when I want to rotate that bump map, it obviously all turns to crap :D
This is NOT a case where I can just liue about the light angle, I already get all that… What I need (for this pre-processing cleverness) is a way of taking that image above, and effectively rotating the whole thing by a given angle, and working out what all the pixel colors would be in that case. This is an arbitrary rotating value (so not just 90 degrees or whatever). I’ll work it out eventually, but I suck at this, and I bet it’s easy. This isn’t speed-dependent stuff so slow is fine, either pure C++ maths or some shader code.
My current shader code for rendering using the normal map:
float4 normalcolor = tex2Dbias(g_samNormalMapColor, texCoord);
normalcolor.b = 0; //experiment to force only r or g channels
//convert it to +/- 1.0 range
normalcolor *= 2.0f;
normalcolor -= 1.0f;
float3 LightDirection;
LightDirection.x = cos(sprite_angle);
LightDirection.y = sin(sprite_angle);
LightDirection.z = 0;
float dot_prod = dot(LightDirection, normalcolor);
Thats just lovely, and obvioously I could lie about the angle I put in, but then how do I get the red and green out of it?