I’ve been testing a bit of a worst case situation for the battle screen in the game where there are just TONS of fighters, and they all have contrails and running lights and you try to see as many of them as possible onscreen.
Not surprisingly the game chugs pretty slowly during that. I’ve been looking at ways to speed the rendering up and have realised something I haven’t been doing enough of is lazy execution.
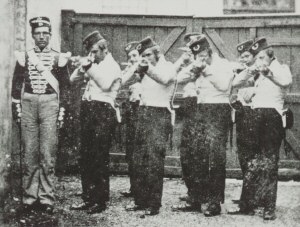
So what is lazy execution in non coding terms?
Imagine your program is designed to tell a robot how to cook (hey why not?). You might have a bit of code that says “Go to the fridge and grab some butter”. That involves a ton of steps, it would involve moving towards the fridge, locating the door, opening the door, removing the butter, etc.
So what you probably do is write some code like this:
FetchItemFromFridge(item)
{
Find fridge
Open Door
Take Item(item)
Close Door
}
And all is lovely and wonderful. Now later on in the programs development you realise you need to do that a lot of items, so your code in another area of the game looks like this:
FetchItemFromFridge(butter);
FetchItemFromFridge(milk);
FetchItemFromFridge(ketchup)
Weird recipe huh? Anyway. this sort of code gets written a lot, especially on big games, because the guy writing the fetchitem code is a different guy to the one writing the recipe code. Often recipe-coder has no idea what happens inside the fetchitem code, so he can’t see any reason to not call it multiple times.
Of course, the sensible re-written code is this:
Find fridge
Open Door
TakeItem(butter)
TakeItem(milk)
TakeItem(ketchup)
Close Door
Now the downside is, that’s a pain to code, because you need to be aware of the circumstances and write code in each case to handle it. This is what lazy execution solves.
Lazy execution means that when I write the code that says “open door”, I don’t actually open the door at all. I just make a mental note that someone wanted the door opened, for reasons we can only speculate about. The good news is, if someone else asks to open the door immediately afterwards, I don’t need to do anything at all.
The real ‘action’ happens when someone calls the ‘takeitem’ code. At that point, the clever lazy stuff says “whoah! you cant take an item without the door being open, I better open that now. At this point, the previous instructions that had been ignored actually get put into action.
The drawback is that the fridge door close needs to be lazy too, which would impact on your carbon emissions :D
Obviously its more complex than that. Incidentally, this is how a lot of directx works. When you call SetRenderState or SetTexture, sod all happens. It only actually does anything if you render something.
The really good news about using lazy code, is that you can optimise anyway all redundancy. In my case the pseudo code causing some issues was stuff like this:
LockVertexBuffer
for each particle
CopyToVertexBuffer
UnlockVertexBuffer
By making the Lock() lazy, the lock is never called (nor is unlock) in cases where no particle needs to be drawn. On big maps, with most of them off screen, this is quite common.