It’s no good doing a game called gratuitous space battles without serious ship explosions. My current benchmark explosion is this one from revenge of the sith:
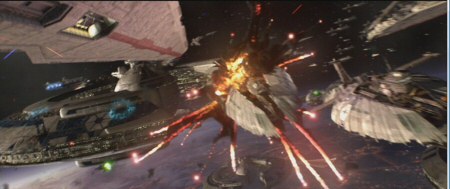
I’ve watched it hundreds of times, and taken about 30 still frame captures from the DVD to use as reference. I have quite a complex particle and effects system for GSB, and it’s coming along nicely. Currently, the average cruiser will have 17 distinct events as it explodes, not counting the release of escape pods
Those 17 events are scripted like this:
[explosions]
1 = 0,128,80,EXP_FRIGATE
2 = 11,97,149,EXP_DESTRUCTION_PLACED
3 = 20,199,205,EXP_DESTRUCTION_PLACED
4 = 20,196,238,EXP_DESTRUCTION_PLACED
5 = 101,108,41,EXP_DESTRUCTION_PLACED
6 = 120,143,163,EXP_DESTRUCTION_PLACED
7 = 200,47,189,EXP_FRIGATEBREAKUP
8 = 210,0,0,EXP_STARTBREAKUP
9 = 270,128,128,EXP_CRUISERDEBRIS
10 = 274,53,226,EXP_DESTRUCTION_PLACED
11 = 290,59,229,EXP_DESTRUCTION_PLACED
12 = 310,171,209,EXP_DESTRUCTION_PLACED
13 = 388,61,204,EXP_DESTRUCTION_PLACED
14 = 400,128,175,EXP_FRIGATEBREAKUP
15 = 400,128,175,EXP_PLUMES
16 = 400,128,128,EXP_BLASTGLARE
17 = 900,128,128,EXP_ANGLED_DEBRIS
They relate to particle effects and other graphics. So there are a number of different particle emitters called from different points on the hull, then at 210 milliseconds after detonation the sprite starts to tessellate into pieces. At the same point, the damaged hulk texture starts to cross fade into existence behind it, and the hulk components start to drift. At 270 milliseconds a shower of permanent debris is spawned. At 400 milliseconds, plumes of wandering particles appear, accompanied by a bright flickering white glare which also generates a shockwave blasting existing debris out of the way. A final shower of temporary particle system debris is triggered right at the end.
This is all configured per-ship-hull, so different ships can detonate in different patterns and styles.
It looks cool :D