EDIT: I’ve fixed it. It’s my own dumbassness. I was setting the sprite_angle variable in the shader AFTER the shader had started, and it was presumably never being set, and populated with garbage (or the ship beforehand) it works a charm now :D
Right so, despite being good at AI coding and optimisation, i suck at this clever stuff you people call ‘3D math’. I was in the pub that day of school. So I enlist you, the all-knowing internet to explain to me like a child what I am doing wrong. Here are 3 images:
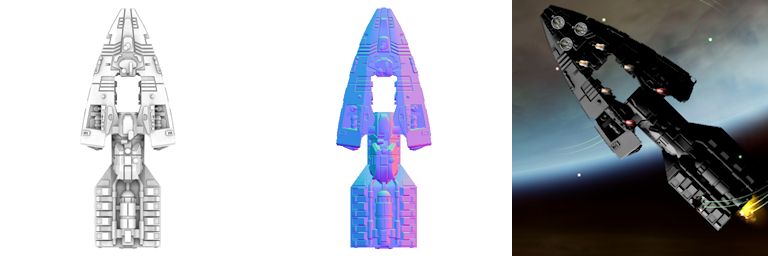
The left is just the ship, the middle is a normal map thingy (thanks to charles!) and the right is what it looks like on screen given a single light source. The end result is exactly what I wanted, and there is much rejoicing… BUT. It’s screwed up, the position of the light source is wrong, and seemingly random, and sometimes jumps and cycles all over the place. I reckon I have everything sorted except the shader, which is an fx file as follows:
sampler2D g_samSrcColor : register( s0);
sampler2D g_samNormalMapColor: register( s1);
float sprite_angle : register(C0);
float4 NormalMap( float2 texCoord : TEXCOORD0 ) : COLOR0
{
//get the value of the normal at this texturecoord
float3 normalcolor = tex2D(g_samNormalMapColor, texCoord);
//convert it to +/- 1.0 range
normalcolor *= 2.0f;
normalcolor -= 1.0f;
float3 LightDirection;
LightDirection.x = sin(sprite_angle);
LightDirection.y = cos(sprite_angle);
LightDirection.z = 0;
float dot_prod = dot(LightDirection, normalcolor);
//apply as a tint to the final pixel
float4 original = tex2D(g_samSrcColor, texCoord);
float4 final = original;
final.r *= dot_prod;
final.b *= dot_prod;
final.g *= dot_prod;
return final;
}
As an idiot, I’m not really sure what I am doing here. What I *think* I’m doing is this: I am drawing a sprite which has a separate normal map (g_samNormalMapColor) I also pass in the current angle of the sprite. I sample the color of the normal map, and convert it into the required range. I then convert the sprites angle into a light direction vector (probably wrongly) and I then do some magic which kids call ‘dot’ which I’m guessing gives me the brightness of the pixel given the angle and the default light direction. I then multiply the original texture color by this brightness to tint my final rendered sprite. Yay.
it’s something clever to do with angles and vectors and stuff isn’t it? explain it to me like I’m an idiot :D
And yeah…I’m playing about with Gratuitous Space Battles. It’s a Sunday morning, don’t read too much into it :D